hello friends welcome to my blog in this blog we learn about how to make pixel led wireless display using the android app
Parts required
- I used the following parts in this project
- pixel led – https://amzn.to/3dJe0s4
- Arduino UNO – https://amzn.to/2WDwGEh
- SMPS – https://amzn.to/2zIjuEM
- HCO5 Bluetooth – https://amzn.to/3fFo9HO
Wiring
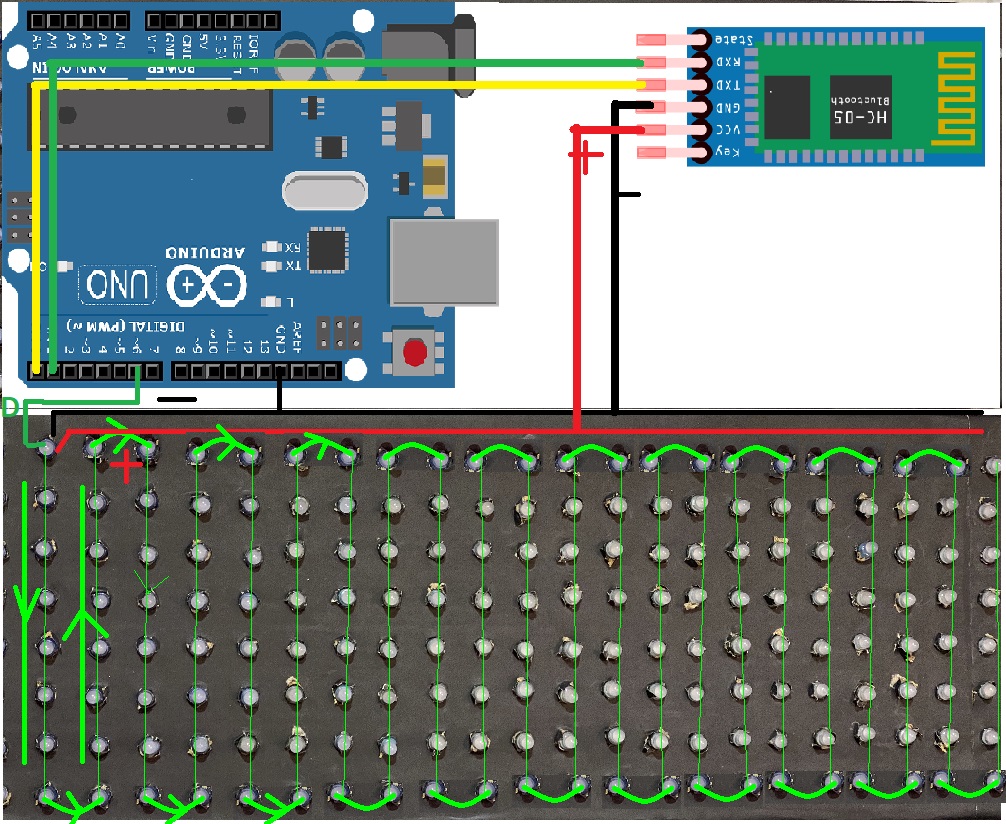
Android app
download app – https://bit.ly/32khLlw
download Arduino library – https://drive.google.com/file/d/1VK1yg1hpBcTI9zC48Yir4lYii5kidkNu/view?usp=sharing
Code for How to make pixel led wireless display
#include <Adafruit_GFX.h>
#include <Adafruit_NeoMatrix.h>
#include <Adafruit_NeoPixel.h>
#include <SoftwareSerial.h>
#include <EEPROM.h>
#define PIN 6
#define EEPROM_MIN_ADDR 0
#define EEPROM_MAX_ADDR 100
#define LEN 450
const String defaultText = " Electronics Ravi ";
// temp variable for storing the displayed text
String in = defaultText;
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(25, 8, PIN,
NEO_MATRIX_TOP + NEO_MATRIX_LEFT +
NEO_MATRIX_COLUMNS + NEO_MATRIX_ZIGZAG,
NEO_GRB + NEO_KHZ800);
SoftwareSerial BTserial(0,1); // RX | TX
const uint16_t colors[] = {
matrix.Color(255, 0, 0),
matrix.Color(0, 255, 0),
matrix.Color(255, 255, 0),
matrix.Color(0, 0, 255),
matrix.Color(255, 0, 255),
matrix.Color(0, 255, 255)};
void setup() {
matrix.begin();
matrix.setTextWrap(false);
matrix.setBrightness(255);
matrix.setTextColor(colors[0]);
randomSeed(analogRead(0));
BTserial.begin(9600);
//Serial.begin(9600);
char chararray[LEN];
if(eeprom_read_string(10, chararray, LEN)) {
//Serial.println(chararray);
in = chararray;
}
}
void loop() {
if(BTserial.available() > 0){
in = BTserial.readString();
char temparray[in.length()+1];
in.toCharArray(temparray, in.length()+1);
if(strstr(temparray, "new") != NULL){
in = strstr(temparray, "new")+3;
char temp[in.length()+1];
in.toCharArray(temp, in.length()+1);
eeprom_write_string(10, temp);
}
else{
in = defaultText;
char temp[in.length()+1];
in.toCharArray(temp, in.length()+1);
eeprom_write_string(10, temp);
}
}
text(random(6));
}
void text(int colorbegin){
int x = matrix.width();
int pass = 0;
while( pass < 3){
matrix.fillScreen(0);
matrix.setCursor(x, 0);
int len = in.length();
matrix.print(in);
if(--x < -len*6) {
x = matrix.width();
pass++;
matrix.setTextColor(colors[(colorbegin+pass)%6]);
}
matrix.show();
delay(80);
}
}
// Input a value 0 to 255 to get a color value.
// The colours are a transition r - g - b - back to r.
uint32_t Wheel(byte WheelPos) {
WheelPos = 255 - WheelPos;
if(WheelPos < 85) {
return matrix.Color(255 - WheelPos * 3, 0, WheelPos * 3);
}
if(WheelPos < 170) {
WheelPos -= 85;
return matrix.Color(0, WheelPos * 3, 255 - WheelPos * 3);
}
WheelPos -= 170;
return matrix.Color(WheelPos * 3, 255 - WheelPos * 3, 0);
}
//Write a sequence of bytes starting at the specified address.
//Returns True if the entire array has been written,
//Returns False if start or end address is not between the minimum and maximum allowed range.
//If False was returned, nothing was written
boolean eeprom_write_bytes(int startAddr, const byte* array, int numBytes) {
int i;
if (!eeprom_is_addr_ok(startAddr) || !eeprom_is_addr_ok(startAddr + numBytes)) return false;
for (i = 0; i < numBytes; i++) {
EEPROM.write(startAddr + i, array[i]);
} return true;
}
//Writes an int value to the specified address.
boolean eeprom_write_int(int addr, int value) {
byte *ptr;
ptr = (byte*)&value;
return eeprom_write_bytes(addr, ptr, sizeof(value));
}
//Reads an integer value at the specified address
boolean eeprom_read_int(int addr, int* value) {
return eeprom_read_bytes(addr, (byte*)value, sizeof(int));
}
//Reads the specified number of bytes at the specified address
boolean eeprom_read_bytes(int startAddr, byte array[], int numBytes) {
int i;
if (!eeprom_is_addr_ok(startAddr) || !eeprom_is_addr_ok(startAddr + numBytes)) return false;
for (i = 0; i < numBytes; i++) {
array[i] = EEPROM.read(startAddr + i);
} return true;
}
//Returns True if the specified address is between the minimum and the maximum allowed range.
//Invoked by other superordinate functions to avoid errors.
boolean eeprom_is_addr_ok(int addr) {
return ((addr >= EEPROM_MIN_ADDR) && (addr <= EEPROM_MAX_ADDR));
}
//Write a string, starting at the specified address
boolean eeprom_write_string(int addr, const char* string) {
int numBytes;
numBytes = strlen(string) + 1;
return eeprom_write_bytes(addr, (const byte*)string, numBytes);
}
//Reads a string from the specified address
boolean eeprom_read_string(int addr, char* buffer, int bufSize) {
byte ch;
int bytesRead;
if (!eeprom_is_addr_ok(addr)) return false;
if (bufSize == 0) return false;
if (bufSize == 1) {
buffer[0] = 0;
return true;
}
bytesRead = 0;
ch = EEPROM.read(addr + bytesRead);
buffer[bytesRead] = ch;
bytesRead++;
while ((ch != 0x00) && (bytesRead < bufSize) && ((addr + bytesRead) <= EEPROM_MAX_ADDR)) {
ch = EEPROM.read(addr + bytesRead);
buffer[bytesRead] = ch;
bytesRead++;
}
if ((ch != 0x00) && (bytesRead >= 1)) buffer[bytesRead - 1] = 0;
return true;
}